For accessing CloudFireStore for Firebase, you need to have Firebase account.
For that you need to go to https://console.firebase.google.com and sign up.
Watch Video Tutorial
# Create a project and give the details
# Once you create the project, Click Add new App in the Project Settings.
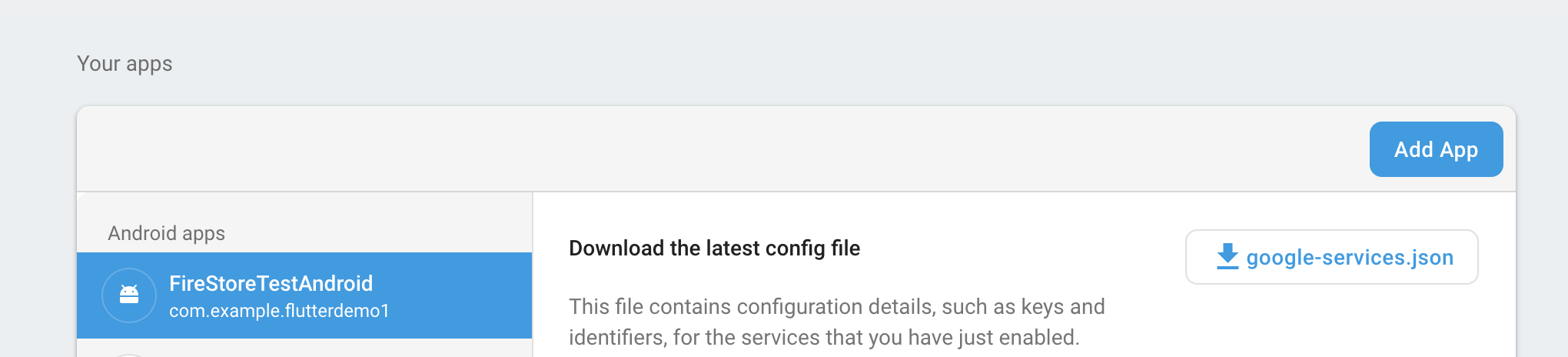
Create new App – Firebase
# Add the Details for your app.
# For Android – Package name can be found in the app-level build.gradle.
# for iOS – Go to General tab for each target in Xcode.
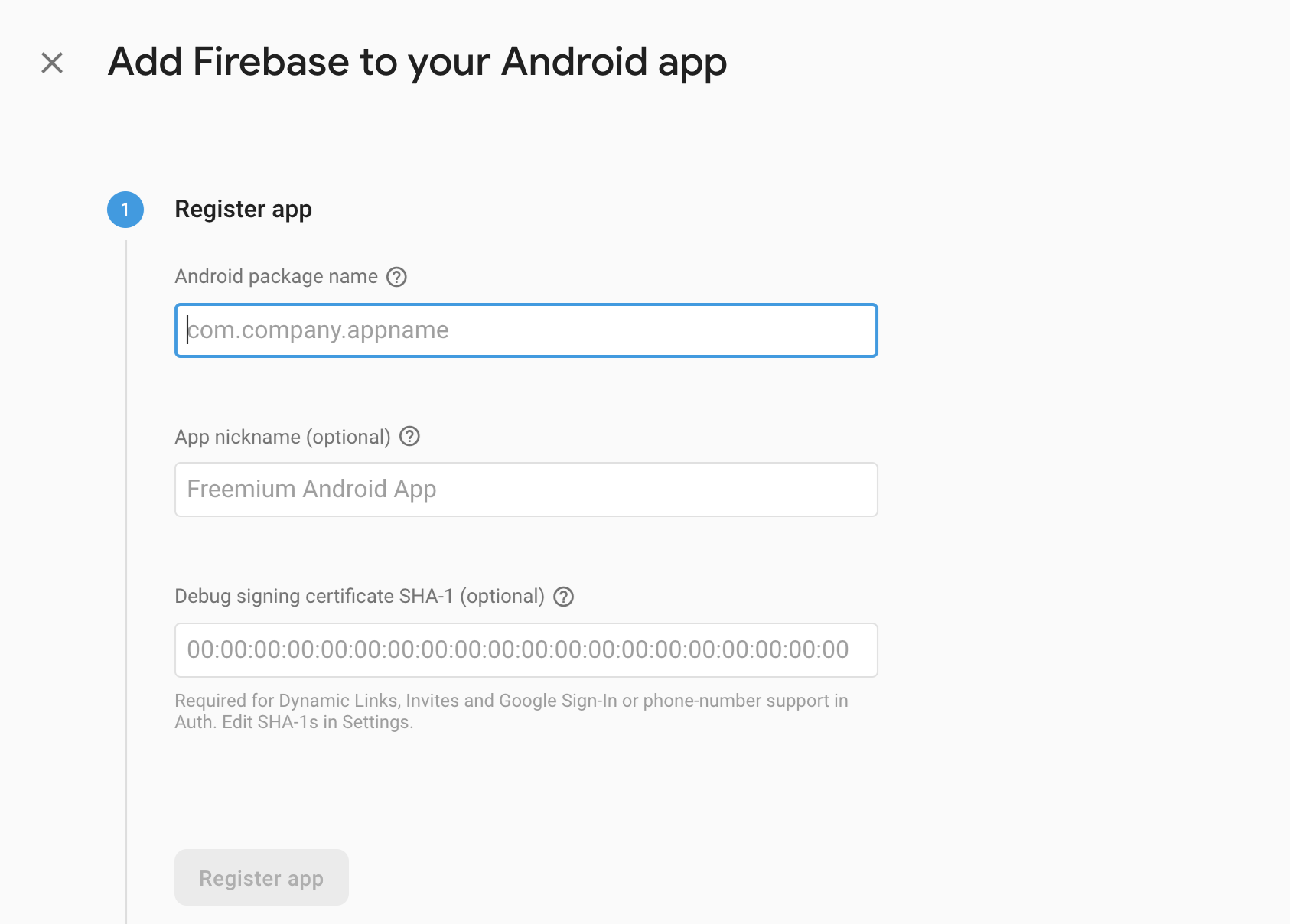
Get the AppID and Register
Both should be same in the case of Flutter.
Once you get the google-services.json & Google-Services-Info.plist for Android and iOS Respectively
For Android copy it into the debug and release folders inside each flavor.
For iOS copy it to the root of the project or corresponding target folders.
# Make sure you have added the configurations need for Android in build.gradle files as per this ink.
https://firebase.google.com/docs/android/setup#add_the_sdk
Once your project and app has created in the Firebase console, we can start working with Flutter code.
Add the package for Flutter
Go to the pubspec.yaml file in the root of your Flutter project and the Flutter package below the dependencies.
dependencies: flutter: sdk: flutter ... cloud_firestore: ^0.9.0+1
Flutter should download the packages for you or run ‘flutter packages get’.
In Firestore, data is stored as collection of documents.
So Lets start the CRUD operations in CloudFireStore.
Get the documents
getUsers() { return Firestore.instance.collection(collectionName).snapshots(); }
Show Users in a List
Widget buildBody(BuildContext context) { return StreamBuilder<QuerySnapshot>( stream: getUsers(), builder: (context, snapshot) { if (snapshot.hasError) { return Text('Error ${snapshot.error}'); } if (snapshot.hasData) { print("Documents ${snapshot.data.documents.length}"); return buildList(context, snapshot.data.documents); } return CircularProgressIndicator(); }, ); } Widget buildList(BuildContext context, List<DocumentSnapshot> snapshot) { return ListView( children: snapshot.map((data) => buildListItem(context, data)).toList(), ); } Widget buildListItem(BuildContext context, DocumentSnapshot data) { final user = User.fromSnapshot(data); return Padding( key: ValueKey(user.name), padding: EdgeInsets.symmetric(vertical: 8.0), child: Container( decoration: BoxDecoration( border: Border.all(color: Colors.grey), borderRadius: BorderRadius.circular(5.0), ), child: ListTile( title: Text(user.name), trailing: IconButton( icon: Icon(Icons.delete), onPressed: () { // delete delete(user); }, ), onTap: () { // update setUpdateUI(user); }, ), ), ); }
Add the Document
addUser() { User user = User(name: controller.text); try { Firestore.instance.runTransaction( (Transaction transaction) async { await Firestore.instance .collection(collectionName) .document() .setData(user.toJson()); }, ); } catch (e) { print(e.toString()); } }
Update Document
update(User user, String newName) { try { Firestore.instance.runTransaction((transaction) async { await transaction.update(user.reference, {'name': newName}); }); } catch (e) { print(e.toString()); } }
Delete User
delete(User user) { Firestore.instance.runTransaction( (Transaction transaction) async { await transaction.delete(user.reference); }, ); }
Complete Source Code
import 'package:flutter/material.dart'; import 'package:cloud_firestore/cloud_firestore.dart'; import 'user.dart'; class FireBaseFireStoreDemo extends StatefulWidget { FireBaseFireStoreDemo() : super(); final String title = "CloudFireStore Demo"; @override FireBaseFireStoreDemoState createState() => FireBaseFireStoreDemoState(); } class FireBaseFireStoreDemoState extends State<FireBaseFireStoreDemo> { // bool showTextField = false; TextEditingController controller = TextEditingController(); String collectionName = "Users"; bool isEditing = false; User curUser; getUsers() { return Firestore.instance.collection(collectionName).snapshots(); } addUser() { User user = User(name: controller.text); try { Firestore.instance.runTransaction( (Transaction transaction) async { await Firestore.instance .collection(collectionName) .document() .setData(user.toJson()); }, ); } catch (e) { print(e.toString()); } } add() { if (isEditing) { // Update update(curUser, controller.text); setState(() { isEditing = false; }); } else { addUser(); } controller.text = ''; } update(User user, String newName) { try { Firestore.instance.runTransaction((transaction) async { await transaction.update(user.reference, {'name': newName}); }); } catch (e) { print(e.toString()); } } delete(User user) { Firestore.instance.runTransaction( (Transaction transaction) async { await transaction.delete(user.reference); }, ); } Widget buildBody(BuildContext context) { return StreamBuilder<QuerySnapshot>( stream: getUsers(), builder: (context, snapshot) { if (snapshot.hasError) { return Text('Error ${snapshot.error}'); } if (snapshot.hasData) { print("Documents ${snapshot.data.documents.length}"); return buildList(context, snapshot.data.documents); } return CircularProgressIndicator(); }, ); } Widget buildList(BuildContext context, List<DocumentSnapshot> snapshot) { return ListView( children: snapshot.map((data) => buildListItem(context, data)).toList(), ); } Widget buildListItem(BuildContext context, DocumentSnapshot data) { final user = User.fromSnapshot(data); return Padding( key: ValueKey(user.name), padding: EdgeInsets.symmetric(vertical: 8.0), child: Container( decoration: BoxDecoration( border: Border.all(color: Colors.grey), borderRadius: BorderRadius.circular(5.0), ), child: ListTile( title: Text(user.name), trailing: IconButton( icon: Icon(Icons.delete), onPressed: () { // delete delete(user); }, ), onTap: () { // update setUpdateUI(user); }, ), ), ); } setUpdateUI(User user) { controller.text = user.name; setState(() { showTextField = true; isEditing = true; curUser = user; }); } button() { return SizedBox( width: double.infinity, child: OutlineButton( child: Text(isEditing ? "UPDATE" : "ADD"), onPressed: () { add(); setState(() { showTextField = false; }); }, ), ); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), actions: <Widget>[ IconButton( icon: Icon(Icons.add), onPressed: () { setState(() { showTextField = !showTextField; }); }, ), ], ), body: Container( padding: EdgeInsets.all(20.0), child: Column( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.start, children: <Widget>[ showTextField ? Column( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.start, children: <Widget>[ TextFormField( controller: controller, decoration: InputDecoration( labelText: "Name", hintText: "Enter name"), ), SizedBox( height: 10, ), button(), ], ) : Container(), SizedBox( height: 20, ), Text( "USERS", style: TextStyle(fontSize: 20, fontWeight: FontWeight.w900), ), SizedBox( height: 20, ), Flexible( child: buildBody(context), ), ], ), ), ); } }
Source Code
You can get the complete source code from here.