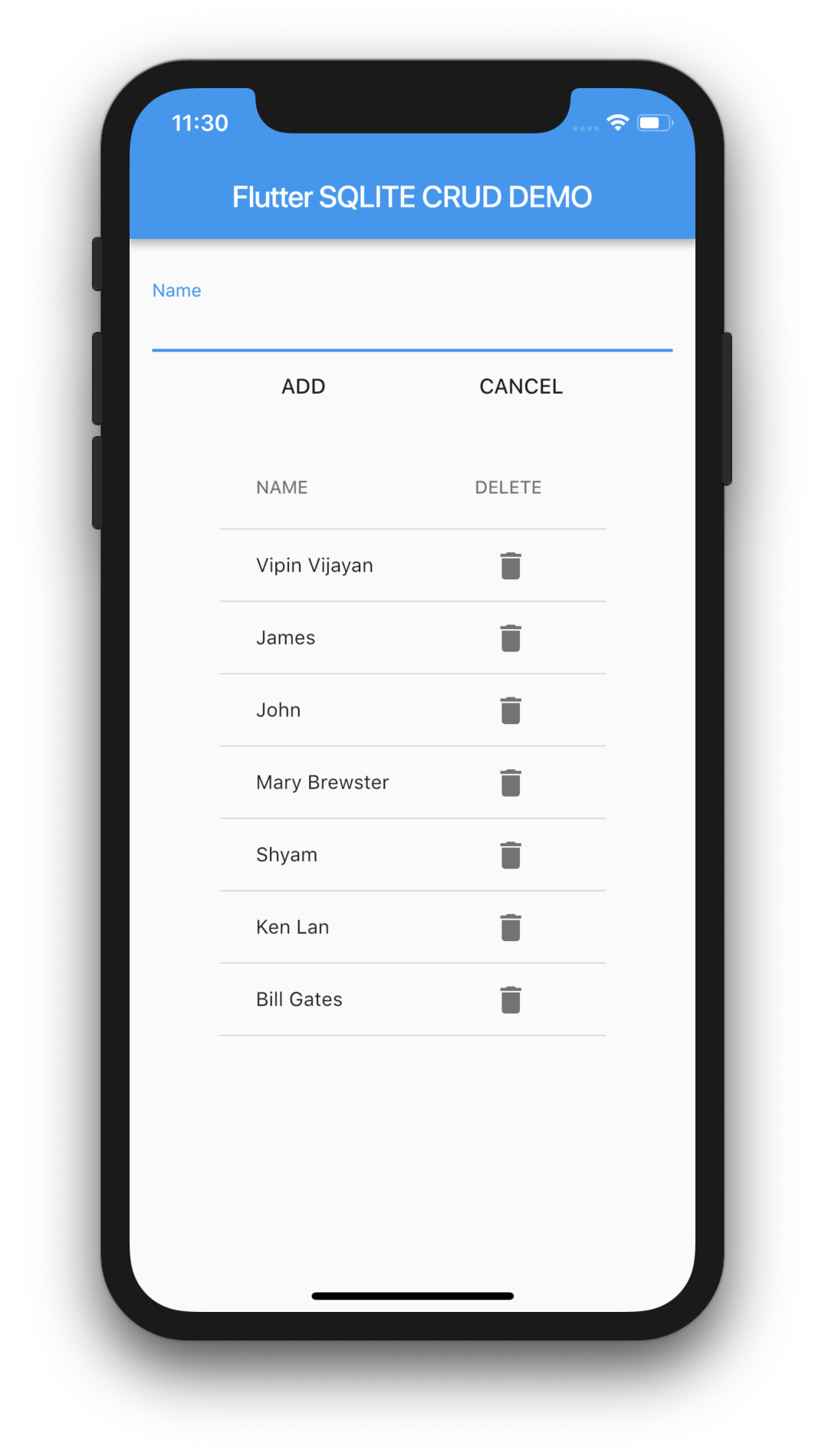
sqlite flutter
Watch Video Tutorial
Add Dependency
SQFlite is a Flutter library for doing local Database Operations.
You can download it from here.
To Integrate SQFlite library in your project
In your flutter project add the dependency:
dependencies: ... sqflite: any
You can download the sample project from here.
employee.dart
class Employee { int id; String name; Employee(this.id, this.name); Map<String, dynamic> toMap() { var map = <String, dynamic>{ 'id': id, 'name': name, }; return map; } Employee.fromMap(Map<String, dynamic> map) { id = map['id']; name = map['name']; } }
Database Utils
Here is a sample DB Utility file.
DBHelper.dart
import 'dart:async'; import 'dart:io' as io; import 'package:path/path.dart'; import 'package:sqflite/sqflite.dart'; import 'package:path_provider/path_provider.dart'; import 'employee.dart'; class DBHelper { static Database _db; static const String ID = 'id'; static const String NAME = 'name'; static const String TABLE = 'Employee'; static const String DB_NAME = 'employee1.db'; Future<Database> get db async { if (_db != null) { return _db; } _db = await initDb(); return _db; } initDb() async { io.Directory documentsDirectory = await getApplicationDocumentsDirectory(); String path = join(documentsDirectory.path, DB_NAME); var db = await openDatabase(path, version: 1, onCreate: _onCreate); return db; } _onCreate(Database db, int version) async { await db .execute("CREATE TABLE $TABLE ($ID INTEGER PRIMARY KEY, $NAME TEXT)"); } Future<Employee> save(Employee employee) async { var dbClient = await db; employee.id = await dbClient.insert(TABLE, employee.toMap()); return employee; /* await dbClient.transaction((txn) async { var query = "INSERT INTO $TABLE ($NAME) VALUES ('" + employee.name + "')"; return await txn.rawInsert(query); }); */ } Future<List<Employee>> getEmployees() async { var dbClient = await db; List<Map> maps = await dbClient.query(TABLE, columns: [ID, NAME]); //List<Map> maps = await dbClient.rawQuery("SELECT * FROM $TABLE"); List<Employee> employees = []; if (maps.length > 0) { for (int i = 0; i < maps.length; i++) { employees.add(Employee.fromMap(maps[i])); } } return employees; } Future<int> delete(int id) async { var dbClient = await db; return await dbClient.delete(TABLE, where: '$ID = ?', whereArgs: [id]); } Future<int> update(Employee employee) async { var dbClient = await db; return await dbClient.update(TABLE, employee.toMap(), where: '$ID = ?', whereArgs: [employee.id]); } Future close() async { var dbClient = await db; dbClient.close(); } }
Implementation Widget
This is the screen that implements the above functions.
import 'package:flutter/material.dart'; import 'employee.dart'; import 'dart:async'; import 'db_helper.dart'; class DBTestPage extends StatefulWidget { final String title; DBTestPage({Key key, this.title}) : super(key: key); @override State<StatefulWidget> createState() { return _DBTestPageState(); } } class _DBTestPageState extends State<DBTestPage> { // Future<List<Employee>> employees; TextEditingController controller = TextEditingController(); String name; int curUserId; final formKey = new GlobalKey<FormState>(); var dbHelper; bool isUpdating; @override void initState() { super.initState(); dbHelper = DBHelper(); isUpdating = false; refreshList(); } refreshList() { setState(() { employees = dbHelper.getEmployees(); }); } clearName() { controller.text = ''; } validate() { if (formKey.currentState.validate()) { formKey.currentState.save(); if (isUpdating) { Employee e = Employee(curUserId, name); dbHelper.update(e); setState(() { isUpdating = false; }); } else { Employee e = Employee(null, name); dbHelper.save(e); } clearName(); refreshList(); } } form() { return Form( key: formKey, child: Padding( padding: EdgeInsets.all(15.0), child: Column( mainAxisAlignment: MainAxisAlignment.center, mainAxisSize: MainAxisSize.min, verticalDirection: VerticalDirection.down, children: <Widget>[ TextFormField( controller: controller, keyboardType: TextInputType.text, decoration: InputDecoration(labelText: 'Name'), validator: (val) => val.length == 0 ? 'Enter Name' : null, onSaved: (val) => name = val, ), Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ FlatButton( onPressed: validate, child: Text(isUpdating ? 'UPDATE' : 'ADD'), ), FlatButton( onPressed: () { setState(() { isUpdating = false; }); clearName(); }, child: Text('CANCEL'), ) ], ), ], ), ), ); } SingleChildScrollView dataTable(List<Employee> employees) { return SingleChildScrollView( scrollDirection: Axis.vertical, child: DataTable( columns: [ DataColumn( label: Text('NAME'), ), DataColumn( label: Text('DELETE'), ) ], rows: employees .map( (employee) => DataRow(cells: [ DataCell( Text(employee.name), onTap: () { setState(() { isUpdating = true; curUserId = employee.id; }); controller.text = employee.name; }, ), DataCell(IconButton( icon: Icon(Icons.delete), onPressed: () { dbHelper.delete(employee.id); refreshList(); }, )), ]), ) .toList(), ), ); } list() { return Expanded( child: FutureBuilder( future: employees, builder: (context, snapshot) { if (snapshot.hasData) { return dataTable(snapshot.data); } if (null == snapshot.data || snapshot.data.length == 0) { return Text("No Data Found"); } return CircularProgressIndicator(); }, ), ); } @override Widget build(BuildContext context) { return new Scaffold( appBar: new AppBar( title: new Text('Flutter SQLITE CRUD DEMO'), ), body: new Container( child: new Column( mainAxisAlignment: MainAxisAlignment.start, mainAxisSize: MainAxisSize.min, verticalDirection: VerticalDirection.down, children: <Widget>[ form(), list(), ], ), ), ); } }
Source Code
Get the complete source code from here.
Hi Vipin,
I am new to Flutter and dart language, i am trying to achieve the below
1. Have to create the custom expandable list ( This will be dynamic based on the server response )
2. Inside header, body contains multiple item (with editfield and radio butttons) again this will also be dynamic based on server response
3. Have to take each input data and store it
Please help me out in this and will be appreciated
Here is my tutorial on Expandable list.
http://www.coderzheaven.com/2019/07/14/expanded-multi-level-list-in-flutter-for-android-and-ios/
Watch Video Tutorial here
https://www.youtube.com/watch?v=k7ttdHmpqEs
Pingback: Flutter DataTable + MySQL – MOBILE PROGRAMMING
Hi James, I m using the code to try to make it work, it works fine but no matter what I do it shows null in name.
If I add, it adds but still shows null
To test that I tried adding several records from a local json, I follow the code and in inserts the records but if
I print this:
if (maps.length > 0) {
for (int i = 0; i < maps.length; i++) {
employees.add(Employee.fromMap(maps[i]));
print('employee added');
print(Employee.fromMap(maps[i]));
}
}
It shows
Instance of 'Employee'
You are printing employee object. use maps[i].name etc.
Pingback: #Google's Flutter Tutorial - SQLite CRUD Operations (coderzheaven.com) - TutsFx